Develop software to help the small bookshop owner to catalog & sell online.
New Project started 2021
-
Stevyn
- SysOp
- Posts:1776
- Joined:Mon Nov 09, 2009 10:03 am
- Location:Japan
-
Contact:
Google Image Search extract tool
Post
by Stevyn » Fri Aug 20, 2021 9:03 am
Google Image Search extract tool
you can simply extract first image URL from Google search results using JSOUP.
https://jsoup.org/
For example: Code to retrieve image URL of the first thumbnail:
Code: Select all
public static String FindImage(String question, String ua) {
String finRes = "";
try {
String googleUrl = "https://www.google.com/search?tbm=isch&q=" + question.replace(",", "");
Document doc1 = Jsoup.connect(googleUrl).userAgent(ua).timeout(10 * 1000).get();
Element media = doc1.select("[data-src]").first();
String finUrl = media.attr("abs:data-src");
finRes= "<a href=\"http://images.google.com/search?tbm=isch&q=" + question + "\"><img src=\"" + finUrl.replace(""", "") + "\" border=1/></a>";
} catch (Exception e) {
System.out.println(e);
}
return finRes;
}
source
https://stackoverflow.com/questions/340 ... -availableContact me directly: Ironfeatherbooks (@) gmail.com
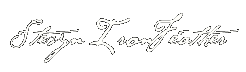
-
Stevyn
- SysOp
- Posts:1776
- Joined:Mon Nov 09, 2009 10:03 am
- Location:Japan
-
Contact:
Post
by Stevyn » Fri Aug 20, 2021 9:07 am
Get Google Search Images using php
You could use the PHP Simple HTML DOM library for this:
Code: Select all
<?php
include "simple_html_dom.php";
$search_query = "ENTER YOUR SEARCH QUERY HERE";
$search_query = urlencode( $search_query );
$html = file_get_html( "https://www.google.com/search?q=$search_query&tbm=isch" );
$image_container = $html->find('div#rcnt', 0);
$images = $image_container->find('img');
$image_count = 10; //Enter the amount of images to be shown
$i = 0;
foreach($images as $image){
if($i == $image_count) break;
$i++;
// DO with the image whatever you want here (the image element is '$image'):
echo $image;
}
This will print a specific number of images (number is set in '$image_count').
http://simplehtmldom.sourceforge.net/
source
https://stackoverflow.com/questions/164 ... -using-php
-------------------------
more examples
http://256cats.com/how-to-scrape-google ... -and-curl/
Web Scrapping in PHP Using Simple HTML DOM Parser :
https://www.geeksforgeeks.org/web-scrap ... om-parser/Contact me directly: Ironfeatherbooks (@) gmail.com
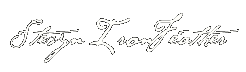